updated 21 september 2012 for blender 2.6.
Hoping to figure this thing out in more detail, here's a snippet that seems to work. It also gives most basic insight into how to translate a Vector using Euler (transform?)
Hoping to figure this thing out in more detail, here's a snippet that seems to work. It also gives most basic insight into how to translate a Vector using Euler (transform?)
import bpy from mathutils import Vector, Euler myTrans = Vector((0.0,0.0,.5)) myEuler = Euler((0.0, 0.3, 0.0),'XYZ') for i in range(14): myVec = Vector((0.0, 0.3, 0.0)) bpy.ops.mesh.extrude_region_move(TRANSFORM_OT_translate={"value":myTrans}) bpy.ops.transform.rotate(value=(0.3,), axis=myVec) myTrans.rotate(myEuler)
- Add a new circle
- select the edges
- run the script.
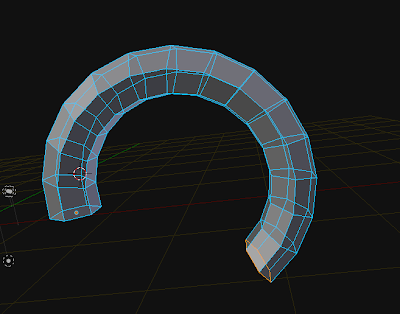
Here is a slight modification, it produces a spiral
import bpy from mathutils import Vector, Euler myTrans = Vector((0.0,0.0,.5)) myEuler = Euler((0.2, 0.3, 0.0),'XYZ') for i in range(34): myVec = Vector((0.2, 0.3, 0.0)) bpy.ops.mesh.extrude_region_move(TRANSFORM_OT_translate={"value":myTrans}) bpy.ops.transform.rotate(value=(0.35,), axis=myVec) myTrans.rotate(myEuler)
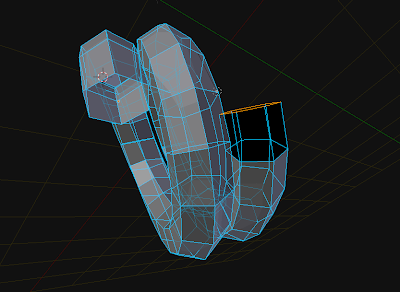
while, the one below produces a more spring like coil.
import bpy from mathutils import Vector, Euler translation = Vector((0.0, 0.0, .5)) for i in range(134): t_dict = {"value": translation} bpy.ops.mesh.extrude_region_move(TRANSFORM_OT_translate=t_dict) bpy.ops.transform.rotate(value=(0.358,), axis=Vector((0.2, 0.3, 0.0))) translation.rotate(Euler((0.2, 0.3, 0.0),'XYZ'))i applied some subsurf to it later.
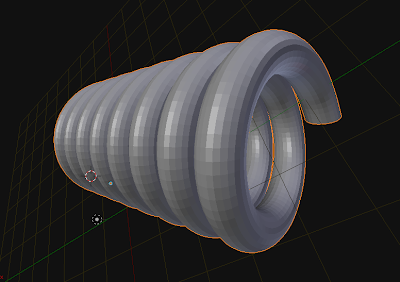